728x90
ArrayList
1. add()
<!DOCTYPE html>
<meta charset="UTF-8">
<title>공장식객체생성2</title>
<script>
var ArrayList = function () {
this.length = 0;
}
ArrayList.prototype.add = function (p_value) {
this[this.length] = p_value;
this.length++;
}
var v_test = new ArrayList();
v_test.add("안녕");
console.log(v_test)
</script>
2. get(), set()
ArrayList.prototype.get = function (p_index) {
return this[p_index];
}
ArrayList.prototype.set = function (p_index) {
this[p_index] = p_index;
}
3. remove, clear
※ remove 배열에서는 값만 사라짐
※ clear 속성의 수만큼 지워줌
ArrayList.prototype.remove = function (p_index) {
delete this[p_index];
this.length--;
}
ArrayList.prototype.clear = function () {
for (var i = 0; i < this.length; i++) {
delete this[i];
}
this.length = 0;
}
※ 더 좋은 remove와 clear ※
더보기
※ 더 좋은 remove와 clear ※
ArrayList.prototype.remove = function (p_index) {
for (var i = p_index; i < (this.length - 1); i++) {
this[i] = this[i + 1];
}
delete this[this.length - 1];
this.length--;
}
ArrayList.prototype.clear = function () {
for (var v_attr in this) {
if (!isNaN(parseInt(v_attr))) {
delete this[v_attr];
}
this.length = 0;
}
this.length = 0;
}
4. size
ArrayList.prototype.size = function (p_index) {
return this.length;
}
완성~
<!DOCTYPE html>
<meta charset="UTF-8">
<title>공장식객체생성2</title>
<script>
var ArrayList = function () {
this.length = 0;
}
ArrayList.prototype.add = function (p_value) {
this[this.length] = p_value;
this.length++;
}
ArrayList.prototype.get = function (p_index) {
return this[p_index];
}
ArrayList.prototype.set = function (p_index) {
this[p_index] = p_index;
}
ArrayList.prototype.remove = function (p_index) {
delete this[p_index];
this.length--;
}
ArrayList.prototype.clear = function () {
for (var i = 0; i < this.length; i++) {
delete this[i];
}
this.length = 0;
}
ArrayList.prototype.size = function (p_index) {
return this.length;
}
</script>
사용해보기
1. add확인
var v_test = new ArrayList();
v_test.add("안녕");
v_test.add("하이");
v_test.add("잘가");
v_test.add("바이");
// add 확인
console.log(v_test);
2. get확인
console.log(v_test.get(3));
3. set확인
v_test.set(3, "수박")
console.log(v_test);
4. size확인
console.log(v_test.size());
5. remove확인
v_test.remove(3);
console.log(v_test);
※ 중간의 내용을 지웠을 때 ※
더보기
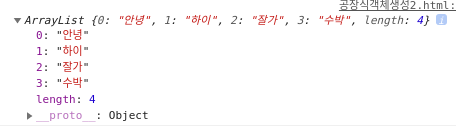
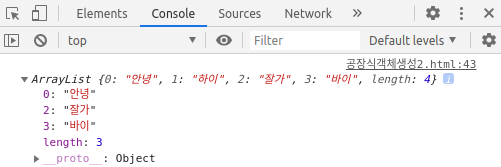
※ 중간의 내용을 지웠을 때 ※
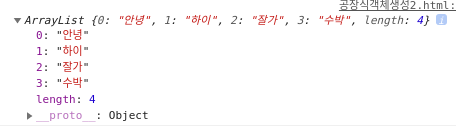
1. remove(1)
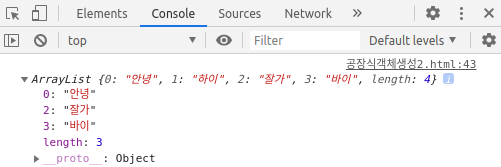
v_test.remove(1);
잘가와 바이가 앞으로 땡겨오지 않았음!!
6. clear확인
v_test.clear();
console.log(v_test);
더보기
<!DOCTYPE html>
<meta charset="UTF-8">
<title>공장식객체생성2</title>
<script>
//자바의 ArrayList를 맹글어 봅시당
// add(value), get(idex), set(index, value),
// remove(index), clear(), size()
var ArrayList = function () {
this.length = 0;
}
ArrayList.prototype.add = function (p_value) {
this[this.length] = p_value;
this.length++;
}
ArrayList.prototype.get = function (p_index) {
return this[p_index];
}
ArrayList.prototype.set = function (p_index, p_value) {
this[p_index] = p_value;
}
ArrayList.prototype.remove = function (p_index) {
//delete this[p_index]; /*delete명령 주의, 배열에서는 값만 사라짐 */
// 중간 index값이 떠버리면 괴로버용
for (var i = p_index; i < (this.length - 1); i++) {
this[i] = this[i + 1];
}
delete this[this.length - 1]; // 땡겨서 마지막 거 지우기
this.length--;
}
ArrayList.prototype.clear = function () {
/* 숫자값 문자열 속성만 삭제 ㅠㅠ NaN이 타입이 Number로 인식ㅠㅠ */
for (var v_attr in this) {
if (!isNaN(parseInt(v_attr))) {
delete this[v_attr];
}
this.length = 0;
}
/* 중간을 지우면 문제가 생기는 코드
for (var i = 0; i < this.length; i++) { // 속성 갯수만큼
delete this[i];
}*/
this.length = 0;
}
ArrayList.prototype.size = function () {
return this.length;
}
/* 확인 잘 되는지? */
var v_test = new ArrayList();
v_test.add("안녕");
v_test.add("하이");
v_test.add("잘가");
v_test.add("바이");
// add 확인
// console.log(v_test);
// get 확인
// console.log(v_test.get(3));
// set 확인
// v_test.set(3, "수박")
// console.log(v_test);
// size 확인
// console.log(v_test.size());
// remove 확인
console.log(v_test);
// v_test.remove(1);
// console.log(v_test);
// clear 확인
v_test.clear();
console.log(v_test);
</script>
'화면구현 > HTML' 카테고리의 다른 글
[lsy만보시오]jQuery의 attr만들기2 (0) | 2020.08.10 |
---|---|
[lsy만보시오] 라이브러리 (0) | 2020.08.10 |
JSON (0) | 2020.08.03 |
ckeditor, cdn (0) | 2020.07.22 |
[php] 파일업로드 (0) | 2020.07.22 |